보ν μ½λ λΆμ
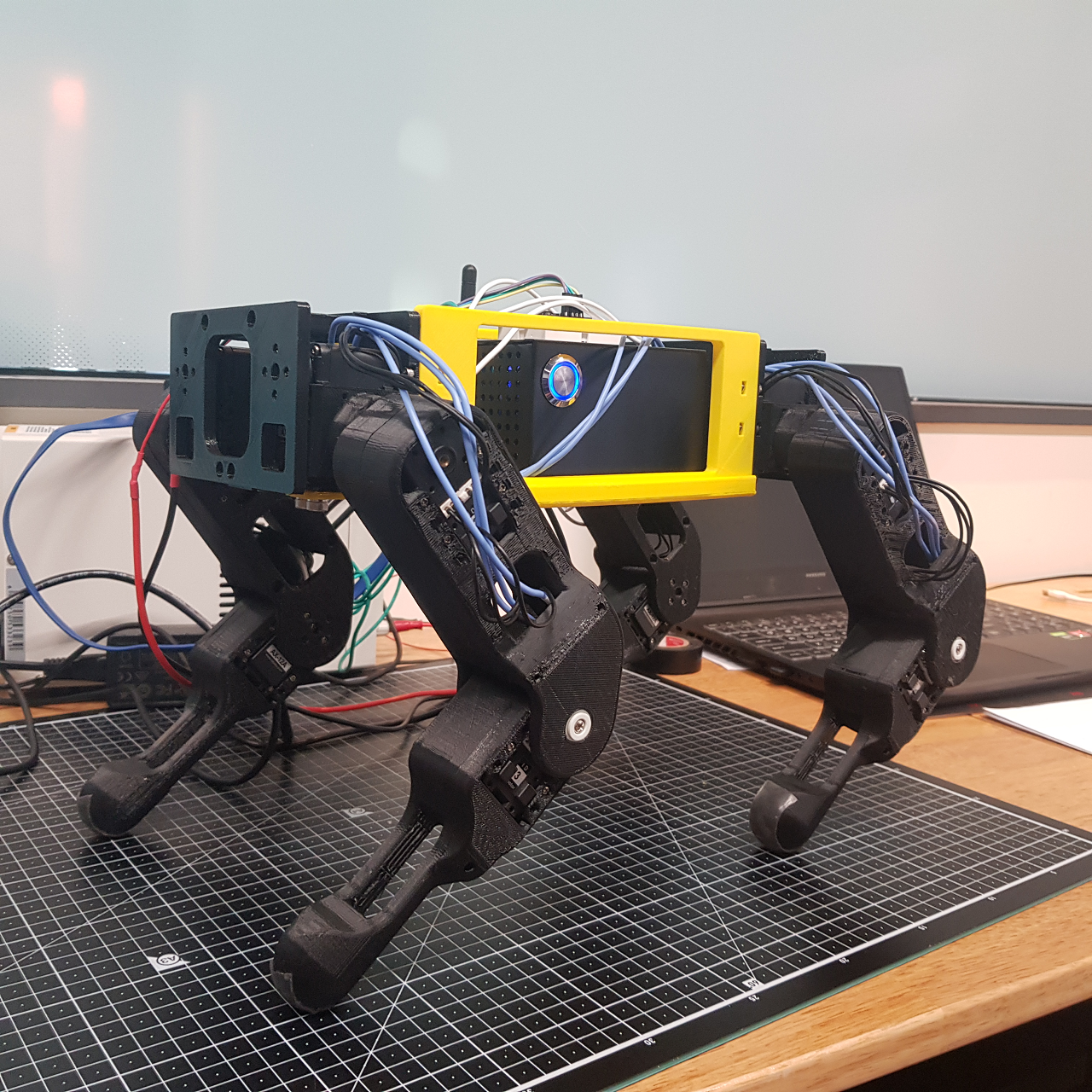
SD_automatic_gait_test.py
μ€μ§μ μΌλ‘ λ‘λ΄μ μμ§μ΄λ μ΅μ’ μ½λλ₯Ό λΆμν΄ λ΄ λλ€.
- λΌμ΄λΈλ¬λ¦¬ import λ° μ΄κΈ° μΈν μ μ§νν©λλ€.
from os import system, name
import sys, os
sys.path.append("..")
sys.path.append(os.path.dirname(os.path.abspath(os.path.dirname(__file__))))
import matplotlib.animation as animation
import numpy as np
import time
import math
import datetime as dt
import keyboard
import random
import board
import busio
import Kinematics.kinematics as kn
import spotmicroai
from SD_servo_controller_test import Dynamixel_Controllers
from multiprocessing import Process
from Common.multiprocess_kb import KeyInterrupt
from Kinematics.kinematicMotion import KinematicMotion, TrottingGait
rtime=time.time()
def reset():
global rtime
rtime=time.time()
spotmicroai
λ μ§κΈκΉμ§ μ°λ¦¬ μ΄ν΄λ³Έ λ‘λ΄μ Kinematics, 보νμ λν λͺ¨λ λ΄μ©μ μ·¨ν©νμ¬ μΆμνν ν΄λμ€μ
λλ€. μμΌλ‘ μ°λ¦¬λ μ΄λ₯Ό ν΅ν΄ λ‘λ΄μ μ μ΄νλ main loopλ₯Ό μ€νμν¬ κ²μ
λλ€.
robot=spotmicroai.Robot(False,False,reset)
- IMU μΌμμ μ΄κΈ° μΈν μ μ§νν©λλ€.
import adafruit_bno055
# BNO055 ( IMU )
i2c_bus0 = busio.I2C(board.SCL_1, board.SDA_1)
sensor = adafruit_bno055.BNO055_I2C(i2c_bus0)
- Dynamixel λͺ¨ν° μ΄κΈ° μΈν μ μ§νν©λλ€.
μ μμ μ μ§ννκΈ° μν΄ USB ν¬νΈμ κΆνμ μ€μ ν΄ μ£Όμ΄μΌ ν©λλ€.
$ cd /dev
$ sudo chmod 775 /dev/ttyUSB0
from dynamixel_sdk import *
# Dynamixel address
ADDR_MX_TORQUE_ENABLE = 24
ADDR_MX_GOAL_POSITION = 30
ADDR_MX_PRESENT_POSITION = 36
PROTOCOL_VERSION = 1.0
BAUDRATE = 1000000
DEVICENAME = '/dev/ttyUSB0'
TORQUE_ENABLE = 1
TORQUE_DISABLE = 0
# setting port and protocol version
portHandler = PortHandler(DEVICENAME)
packetHandler = PacketHandler(PROTOCOL_VERSION)
DXL_controller = Dynamixel_Controllers()
# setting the DXL_Motor
DXL_controller.DynamixelSetting()
spurWidth=robot.W/2+50
stepLength=0
stepHeight=72
# Initial End point X Value for Front legs
iXf=120
walk=False
λ‘λ΄μ 보νκ³Ό κ΄λ ¨λ λ§€κ°λ³μλ₯Ό μ€μ ν©λλ€.
spurWidth
: μ’μ° λ° μ¬μ΄μ λλΉμ ν΄λΉνλ λ§€κ°λ³μμ λλ€.
stepHeight
: 보ν μ λ°μ λ€μ΄μ¬λ¦¬λ λμ΄μ λλ€.
iXf
: λ‘λ΄ μλ°μ μ΄κΈ° x μ’νλ‘, λ¬΄κ² μ€μ¬μ λ°λ₯Έ μμ μ±μ μν΄ μ΅μ ν μ μ¬μ©ν©λλ€.
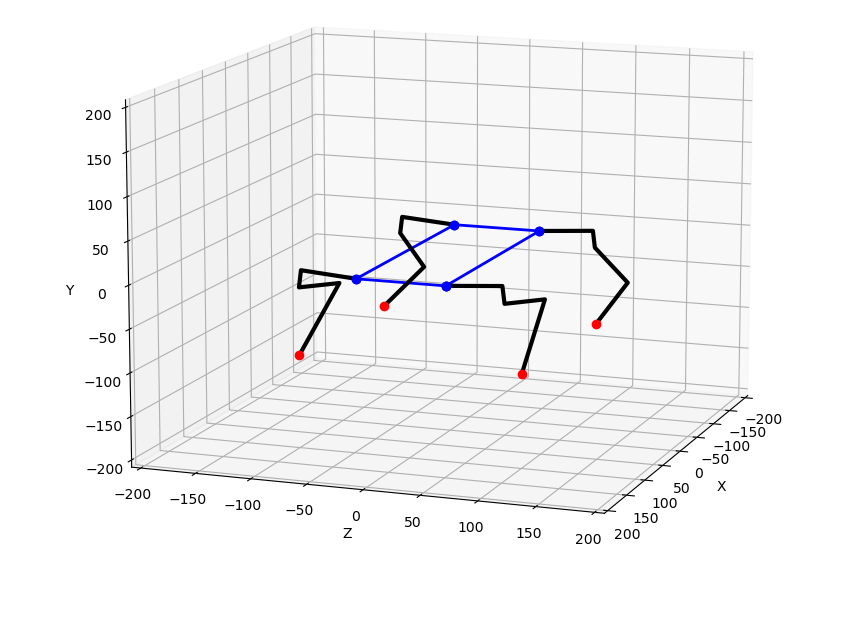
- κ°κ°μ Leg Point (x, y, z μ’ν)λ₯Ό μ§μ ν©λλ€.
Lp = np.array([[iXf, -170, spurWidth, 1], [iXf, -170, -spurWidth, 1],
[-120, -170, spurWidth, 1], [-120, -170, -spurWidth, 1]])
- 보νμ μν ν΄λμ€,
TrottingGait
λ₯Ό μ μΈνλ λͺ¨μ΅μ λλ€.
motion=KinematicMotion(Lp)
resetPose()
trotting=TrottingGait()
Main Loop
λλΆλΆμ λ‘λ΄μ 무ν while loop μμμ μ§μμ μΌλ‘ νΌλλ°±, ꡬλμ λ°λ³΅ν©λλ€.
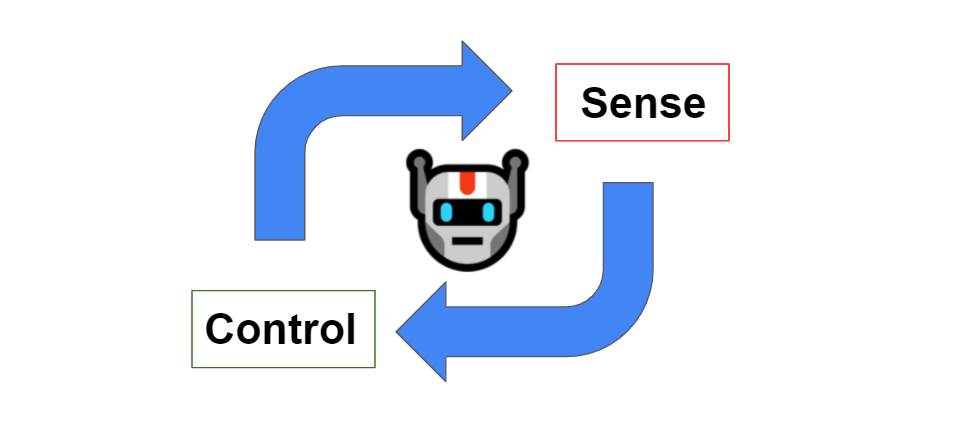
μ§κΈμ κ²½μ° μ°λ¦¬ λ‘λ΄μ
- IMUλ‘ λΆν° μμΉ λ°μ΄ν°λ₯Ό μΌμ±νμ¬
- λ‘λ΄μ΄ λμ΄μ§μ§ μκ² λμμμ΄ μ μ§νλ λ‘μ§μ΄ ꡬλλκ³ μμ΅λλ€.
μ΄λ¬ν κ΄μ μΌλ‘ main loopλ₯Ό λΆμν΄ λ³΄κ² μ΅λλ€. π
- loopκ° λ°λ³΅λ λλ§λ€ ꡬλ μκ°μ΄ μ λ°μ΄νΈ λ©λλ€.
def main(id, command_status):
jointAngles = []
while True:
xr = 0.0
yr = 0.0
# Reset when robot pose become strange
# robot.resetBody()
ir = xr/(math.pi/180)
d = time.time()-rtime
# robot height
height = 30 # 40
- ν€λ³΄λ μ λ ₯μ ν΅ν΄ λ‘λ΄μ κ²½λ‘λ₯Ό μ μ΄νλ λΆλΆμ λλ€.
# calculate robot step command from keyboard inputs
result_dict = command_status.get()
print(result_dict)
command_status.put(result_dict)
# wait 3 seconds to start
if result_dict['StartStepping']:
currentLp = trotting.positions(d-3, result_dict)
robot.feetPosition(currentLp)
else:
robot.feetPosition(Lp)
pitch = -((sensor.euler[1]*math.pi)/180)
yaw = -((sensor.euler[0]*math.pi)/180)
roll = -((sensor.euler[2]*math.pi)/180)
robot.bodyRotation((roll,yaw,pitch))
robot.bodyPosition((0, 0, 0))
IMUλ‘λΆν° λͺΈμ²΄μ κ°λλ₯Ό μ λ¬λ°κ³ , μ΄λ₯Ό spotmicroai
robot classμ bodyRotation
ν¨μμ μ λ¬ν©λλ€.
def bodyRotation(self,rot):
self.rot=rot
def calcIK(self,Lp,angles,center):
(omega,phi,psi)=angles
(xm,ym,zm)=center
...
bodyRotation
ν¨μλ rot λ³μμ λͺΈμ²΄ κ°λ μ 보λ₯Ό μ μ₯νμ¬ μΆν λͺ¨ν° κ°λλ₯Ό κ³μ°ν λ
(calcIK
ν¨μμμ rot λ³μλ₯Ό μ¬μ©ν©λλ€.) λ‘λ΄ λͺΈμ²΄κ° κΈ°μ΄ κ²μ λν 보μμ ν μ μλλ‘ ν©λλ€.
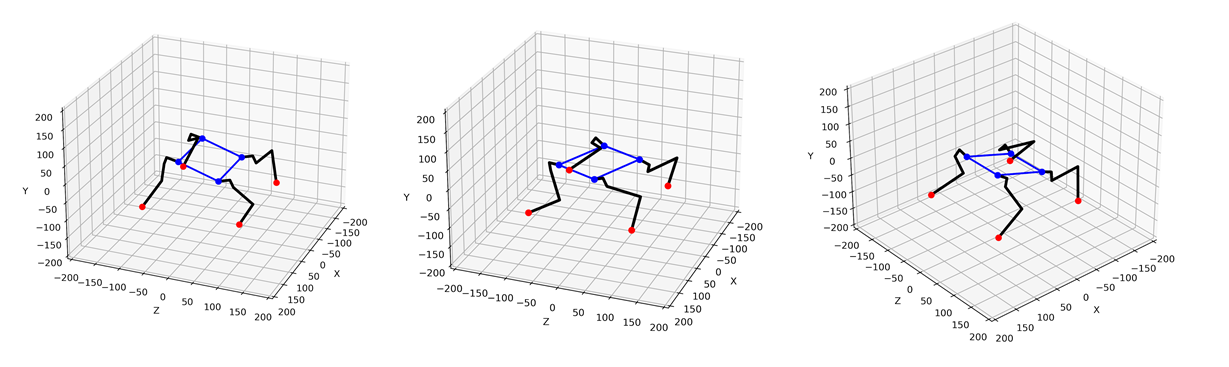
μ¦, λ°λ₯μ΄ κΈ°μΈμ΄μ Έ λ‘λ΄μ λͺΈμ²΄κ° κΈ°μΈμμλ λ€λ¦¬μ μμΈλ₯Ό λ³κ²½νμ¬ λͺΈμ²΄μ κ°λκ° μΈλΆμ μ’νκ³ μ μ₯μμ μΌμ νκ² μ μ§ν μ μλλ‘ ν©λλ€.
- λ€μμΌλ‘, λ‘λ΄μ λͺ¨ν°λ₯Ό μ μ΄νκΈ° μν κ°λλ₯Ό λ°μμ
LaDian
λ³μμ μ μ₯νλ λΆλΆμ λλ€.
⇒ λλ²κΉ μ μν΄ μ΄ λΆλΆμ΄ μ½μμ μΆλ ₯λ©λλ€.
LaDian = robot.getAngle()
print(LaDian)
...
# spotmicroai.py
def step(self):
if (self.useRealTime):
self.t = time.time() - self.ref_time
else:
self.t = self.t + self.fixedTimeStep
# print(self.t)
kp=self.IDkp # p.readUserDebugParameter(self.IDkp)
kd=self.IDkd #p.readUserDebugParameter(self.IDkd)
maxForce=self.IDmaxForce #p.readUserDebugParameter(self.IDmaxForce)
# Calculate Angles with the input of FeetPos,BodyRotation and BodyPosition
self.angles = self.kin.calcIK(self.Lp, self.rot, self.pos)
...
- μ΄ angleμ spotmicroai.py νμΌ μμ step ν¨μμμ μ΄λ£¨μ΄μ§λλ€.
- λͺΈμ²΄μ μμΉ, κ°λ, λ°μ μμΉ μ 보λ₯Ό μ
λ ₯νλ©΄
calIK
ν¨μλ₯Ό ν΅ν΄ μ΄ λμμ ꡬννκΈ° μν κ° κ΄μ λͺ¨ν°μ κ°λκ° κ³μ°λμ΄ κ°±μ λ©λλ€.
λ°μμ¨ λͺ¨ν° κ°λλ₯Ό κ° λ€μ΄λλ―Ήμ μ μ λ¬νλ λΆλΆμ λλ€.
κ·Έλ¬λ©΄ μ΅μ’ μ μΌλ‘ 보ν ν¨ν΄μ λ°λΌ λͺ¨ν°κ° ꡬλμ΄ λμ΄ κ±·λ λμμ μννκ² λ©λλ€. π
if len(LaDian):
# ladian to degree
thetas = DXL_controller.LadianToAngles(LaDian)
# adjust robot servo
Goal_Degree = DXL_controller.AngleToServo(DXLMotor_N)
# degree to dynamixel value
Goal_Position_Value = DXL_controller.DegreeToDXLValue(Goal_Degree)
# Real Actuators
DXL_controller.WriteMotor(DXLMotor_N)
print(" **** Goal Degree **** ")
print(Goal_Degree)
# # Plot Robot Pose into Matplotlib for Debugging
# TODO: Matplotplib animation
# kn.initFK(jointAngles)
# kn.plotKinematics()
robot.step()
consoleClear()
ν€λ³΄λ μ‘°μ’
λ©μΈ μ½λλ₯Ό μ€νν λ€, μ€μ λ‘λ΄μ μ νμ’μ°λ‘ μ΄λμν€κΈ° μν λ°©λ²μ λλ€.
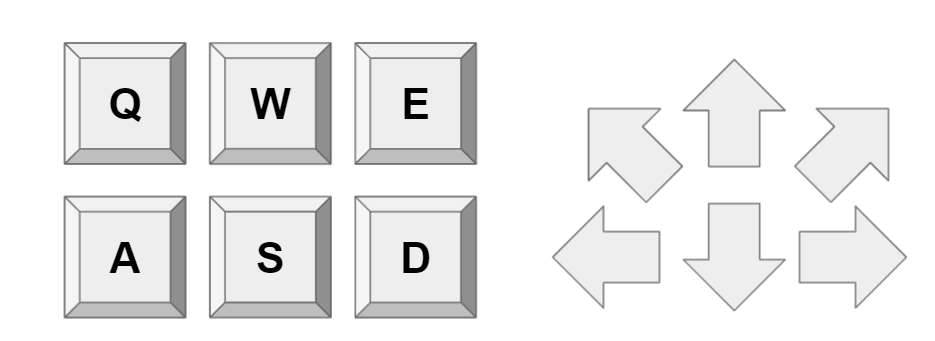
W A S D : κ²μκ³Ό κ°μ΄ μ νμ’μ°λ‘ λ‘λ΄μ μμ§μ΄κ² νλ©°, ν λ² λλ₯Ό λλ§λ€ ν΄λΉ λ°©ν₯μΌλ‘μ μλκ° μ¦κ°νλ λ°©μμ λλ€.
Q E : μκ³λ°©ν₯, λ°μκ³λ°©ν₯μΌλ‘ λ‘λ΄μ νμ + μ΄λμν€κ² λ©λλ€.
μλ₯Ό λ€μ΄
W 10λ²
+S 5λ²μ
λλ₯΄λ©΄ W 5λ² λ§νΌμ μ μ§ μλλ₯Ό κ°κ³ λ‘λ΄μ΄ μμ§μ λλ€.